Implementing Role-Based Access Control in Ruby on Rails
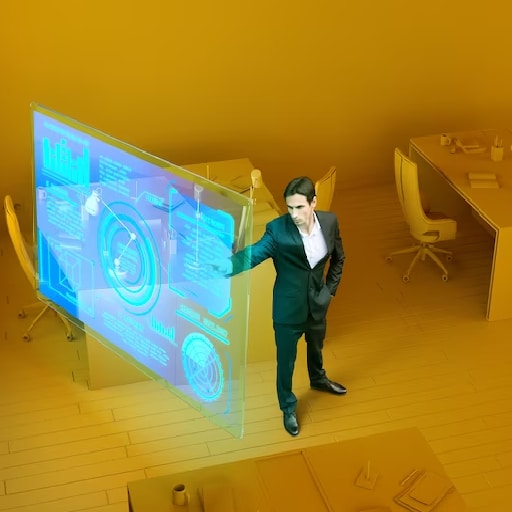
To implement Role-Based Access Control (RBAC) in Ruby on Rails, you can follow these steps:
Step 1: Set up the database tables
Create the necessary database tables to store roles, permissions, and user-role mappings. You can use a migration file to create these tables. For example:
“`ruby
rails generate migration CreateRoles name:string
rails generate migration CreatePermissions name:string
rails generate migration CreateUserRoles user_id:integer role_id:integer
“`
Step 2: Define the models
Create the corresponding models for roles, permissions, and user-role mappings. For example:
“`ruby
class Role < ApplicationRecord
has_many :user_roles
has_many :users, through: :user_roles
end
class Permission < ApplicationRecord
has_and_belongs_to_many :roles
end
class UserRole < ApplicationRecord
belongs_to :user
belongs_to :role
end
“`
Step 3: Set up associations
Define the associations between the User model and the Role model. For example:
“`ruby
class User < ApplicationRecord
has_many :user_roles
has_many :roles, through: :user_roles
end
“`
Step 4: Define authorization methods
Create methods in the User model to check if a user has a specific role or permission. For example:
“`ruby
class User < ApplicationRecord
# …
def has_role?(role_name)
roles.exists?(name: role_name)
end
def has_permission?(permission_name)
roles.joins(:permissions).exists?(permissions: { name: permission_name })
end
end
“`
Step 5: Set up authorization checks
In your controllers or views, use these authorization methods to check if a user has the required role or permission. For example:
“`ruby
class UsersController < ApplicationController
def index
if current_user.has_role?(‘admin’)
# Only admins can access this action
@users = User.all
else
# Redirect or show an error message
redirect_to root_path, alert: ‘You are not authorized to access this page.’
end
end
end
“`
Step 6: Assign roles and permissions to users
In your application, provide a way to assign roles and permissions to users. This can be done through a user interface or by manually updating the database.
These steps provide a basic implementation of RBAC in Ruby on Rails. You can further enhance it by adding more features like role hierarchy, dynamic permissions, and more granular access control.