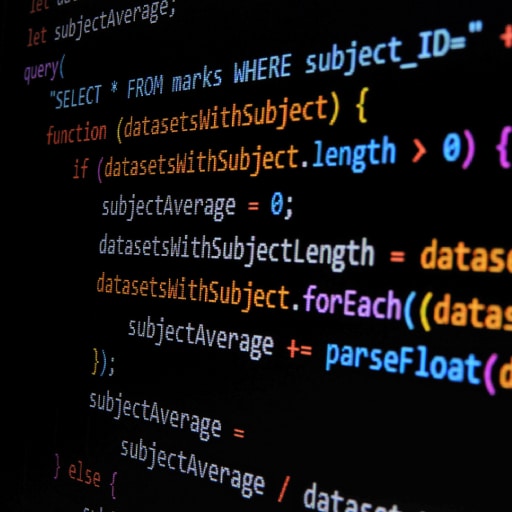
Node.js is a powerful JavaScript runtime that allows you to build scalable and efficient server-side applications. If you’re new to Node.js, this beginner’s guide will help you get started with the basics.
1. Install Node.js:
– Visit the official Node.js website (https://nodejs.org) and download the latest stable version for your operating system.
– Run the installer and follow the instructions to complete the installation.
2. Verify the installation:
– Open a terminal or command prompt and type `node -v`. This command will display the installed version of Node.js. If you see the version number, it means Node.js is successfully installed.
3. Create a new Node.js project:
– Choose a directory where you want to create your project.
– Open a terminal or command prompt and navigate to the chosen directory.
– Run the command `npm init` to initialize a new Node.js project. This command will prompt you to enter some information about your project, such as the name, version, and entry point file. You can press enter to accept the default values for most of the prompts.
4. Install dependencies:
– Node.js uses a package manager called npm (Node Package Manager) to manage dependencies.
– To install a package, run the command `npm install
– The installed packages will be saved in the `node_modules` directory in your project.
5. Create a basic Node.js server:
– Open your preferred code editor and create a new file named `server.js` (or any other name you prefer) in your project directory.
– In `server.js`, require the `http` module by adding the following line of code:
“`javascript
const http = require(‘http’);
“`
– Create a server by using the `createServer` method of the `http` module:
“`javascript
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader(‘Content-Type’, ‘text/plain’);
res.end(‘Hello, World!’);
});
“`
– Start the server by listening on a specific port:
“`javascript
const port = 3000;
server.listen(port, () => {
console.log(`Server running on port ${port}`);
});
“`
6. Run the Node.js server:
– Open a terminal or command prompt and navigate to your project directory.
– Run the command `node server.js` to start the server.
– Open a web browser and visit `http://localhost:3000`. You should see the message "Hello, World!" displayed on the page.
Congratulations! You have successfully created a basic Node.js server. This is just the beginning, and there is a lot more to learn about Node.js. You can explore various frameworks, libraries, and tools to build more complex applications.